Next.js Font Management: A Complete Guide
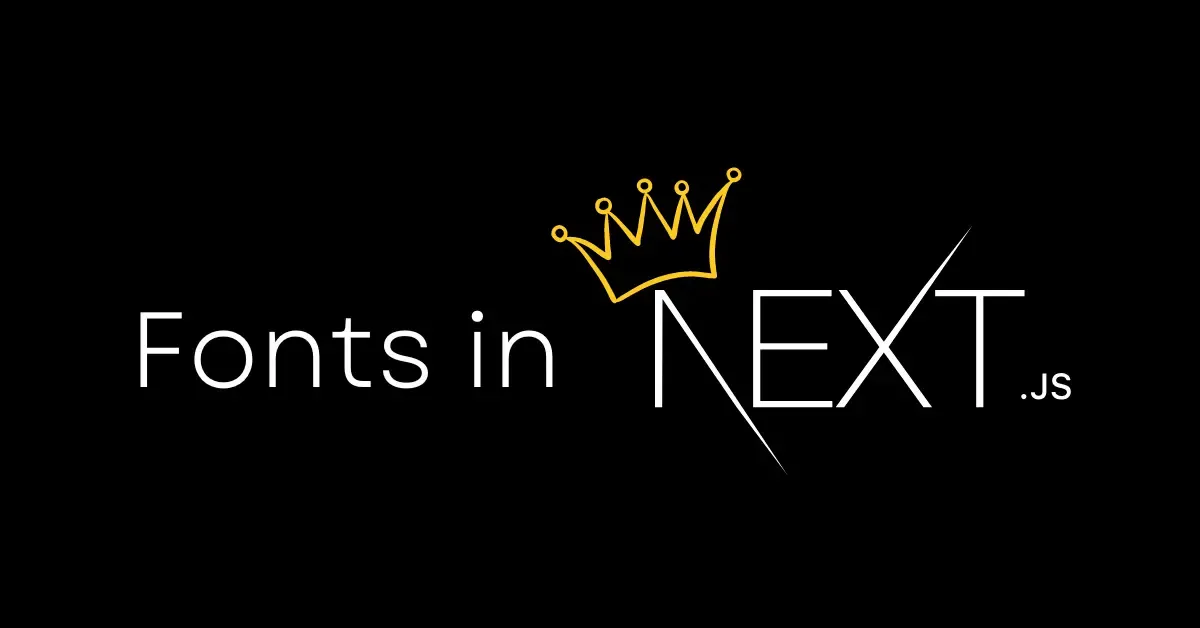
In this toutorial we will learn how to use next.js.
If Your open you layout.tsx
if your are using typescript or layout.jsx
if you are using javascript, you will find this content.
import { Inter } from "next/font/google";
const inter = Inter({ subsets: ["latin"] });
export default function RootLayout({ children,}: Readonly<{ children: React.ReactNode;}>) { return ( <html lang="en" data-theme="light"> <body className={inter.className}>{children}</body> </html> );}
next.js using google font by default, so you can use any google font easly by importing with the same way. for example let us change the font from inter
into roboto
.
import { Roboto } from "next/font/google";
const roboto = Roboto({ subsets: ["latin"] });
export default function RootLayout({ children,}: Readonly<{ children: React.ReactNode;}>) { return ( <html lang="en" data-theme="light"> <body className={roboto.className}>{children}</body> </html> );}
and with the same way we can use any google font that we like.
#Working with Local Fonts
The question now is what if I want to use local font that did not included in google fonts?
That is not a problem, in next.js we can handel local fonts with easy way.
import localFont from "next/font/local";
const myFont = localFont({ src: "./my-font.woff2" });
export default function RootLayout({ children,}: Readonly<{ children: React.ReactNode;}>) { return ( <html lang="en" data-theme="light"> <body className={myFont.className}>{children}</body> </html> );}
#Use Fonts with Tailwind CSS
Until now we learned how to handle fonts in next.js whether its google font or loval font.
But what if we are using tailwind in my project ?
Fortunately we have straighforward way to solve this problem.
#First we should pass the font as a css variable in the layout:
import { Inter } from "next/font/google";
const inter = Inter({ subsets: ["latin"], variable: "--font-inter"});
export default function RootLayout({ children,}: Readonly<{ children: React.ReactNode;}>) { return ( <html lang="en" data-theme="light"> <body className={inter.variable}>{children}</body> </html> );}
#Define the font in tailwind config file
At this time we made our font as a global css variable in our app. This means that we can use it in our tailwind
config file directly.
import type { Config } from "tailwindcss";
const config: Config = { content: [ "./pages/**/*.{js,ts,jsx,tsx,mdx}", "./components/**/*.{js,ts,jsx,tsx,mdx}", "./app/**/*.{js,ts,jsx,tsx,mdx}", ], theme: { extend: { fontFamily: { inter: ["var(--font-inter)"], }, }, },};export default config;
by this change we can now use our font in any component in our project using tailwind classes.
#Using font through tailwind classes
const CustomComponent = () => { return ( <section> <h1 className="font-inter">Hello, World!</h1> </section> );};
In this Toutorial we learned how to work with fonts in next.js with different ways.
Finally I hope you find this toutorial useful and see you in the next toutorial.